After googling, I could not find a checkbox component that somebody else had made or a good tutorial. So below are the steps I did to create a simple checkbox.
I began by adding a UIButton to my view and setting its Type to custom and its background to my unchecked png image with transparent background. Then I implemented the auto generated viewDidLoad method to set my background image if the button state was selected to the checked png image.
- (void)viewDidLoad {
[super viewDidLoad];
[checkButton setBackgroundImage:[UIImage imageNamed:@"checked.png"] forState:UIControlStateSelected];
}
Finally, I attached a changeSeletected method to the button's Touch Up Inside event that changed the selected state of my button.
- (IBAction) changeSelected: (id) sender {
UIButton *button = (UIButton *) sender;
button.selected = !button.selected;
}
I think the final outcome turned out pretty nice.
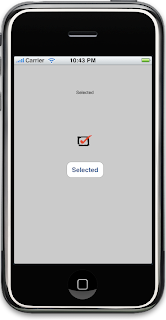
5 comments:
Nice. Thanks. I have a settings screen where real-estate is tight, so this will fit in nicely.
Here, Wyvwyv say that:
"I ended up creating my own UICheckbox control and stole the checkbox graphics from OS X. I subclassed a UIButton and made the button action toggle its own selected state."
But i don't know how to do that.
Pls someone help me.....
thanhbv,
I can speak for Wyvwyv but you can download my sample code at http://juddsolutions.com/downloads/iPhoneCheckbox.zip.
Yes, thank you very much. But why don't you setBackgroundImage: @checked.png forState:Selected right in IB? (you set it programmatically in CheckboxViewController.viewDidLoad()).
I think the solution may like this:
1. create UICheckbox : UIButton
2. override UICheckbox.awakeFromNib and call:
[self addTarget:self action:@selector(checkboxTapped:) forControlEvents:UIControlEventTouchUpInside];
3. Implement UICheckbox.checkboxTapped {self.selected = ! self.selected;}
4. In IB, add our Checkbox control into a view, set backgroundImage for it states
:D but it seems that the UICheckbox.addTarget:action:forControlEvents don't work!
Do you have any idea?
Yes, I found you can set up the checkbox as a custom button in Interface Builder and use only a few lines of code to get it to work. Have a look at my tutorial and source code here, as an alternative.
Post a Comment